dbt jinja: Reuse Code Snippets
In dbt, Jinja (opens in a new tab) is a templating language used alongside SQL to elevate your data transformation workflows. While SQL alone performs data manipulation, Jinja adds a layer of dynamism to your SQL scripts within dbt projects, allowing for conditional logic, loops, and variable management. This combination transforms your project into a robust SQL programming environment.
Key Features of Jinja in dbt
- Control Structures: Incorporate logic like if-conditions and loops directly within SQL queries to handle complex data scenarios.
- Environment Variables: Use variables for dynamic adjustments during production deployments.
- Dynamic SQL Generation: Craft SQL queries on the fly based on previous query results, aiding in tasks such as data aggregation or schema alignment.
Practical Example
Imagine a scenario where you need to generate a report detailing various revenue streams based on different product categories in a sales dataset. Here's how you can use Jinja to dynamically create SQL queries to accommodate multiple categories efficiently:
This Jinja template in dbt dynamically adds subtotal columns for each product category, compiling into a SQL query that performs targeted aggregations for a streamlined analysis of revenue by category.
Macros: Reusable Code Blocks
To further demonstrate the power of macros in dbt, here's an example that calculates a loyalty discount based on customer tenure:
Usage in a model:
This macro makes it easy to apply a tiered discount logic across multiple models, ensuring consistency and reducing the potential for errors while promoting code reusability.
Best Practices
- Simplify Your SQL: While Jinja offers powerful capabilities, using it extensively can make SQL code harder to read and maintain. Balance the use of Jinja to ensure your models remain accessible.
- Macro Efficiency: Before creating a new macro, check if there's an existing one in dbt's community packages like dbt-utils that meets your needs.
- Debugging: Use dbt's compile command to preview the raw SQL generated by your Jinja templates, helping you troubleshoot and refine your code.
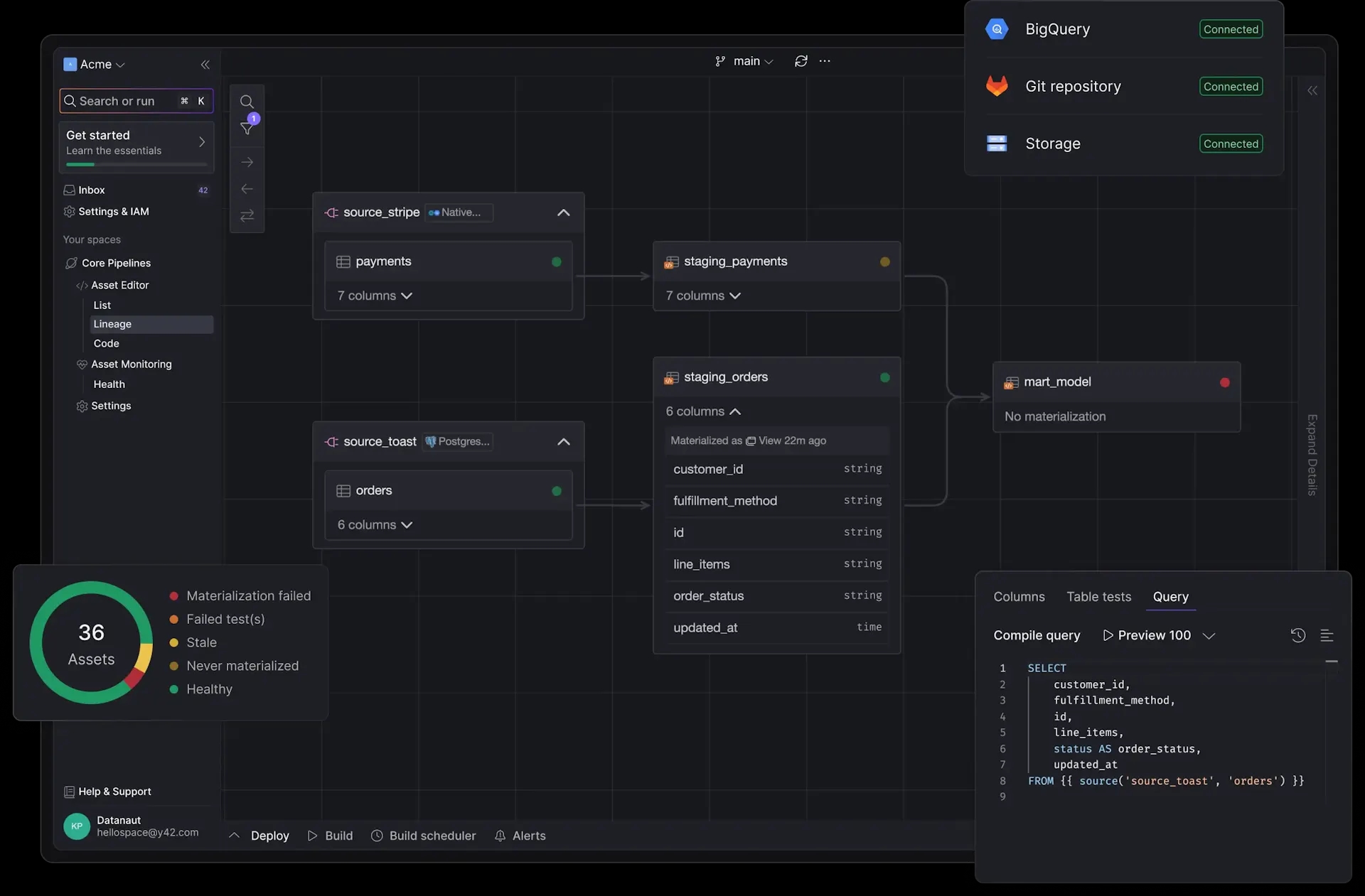
Manage Sources and dbt Models in one place
Build end-to-end pipelines using a single framework.
Get Started