dbt macros: Reuse Code Snippets
dbt macros are code snippets that allow you to write reusable code segments, similar to functions in programming languages.
By leveraging Jinja, dbt’s templating language of choice, you can create macros that simplify and automate repetitive SQL tasks, making your data transformation workflows more efficient and maintainable.
What Are Macros?
A macro in dbt is a reusable piece of code that you can invoke in various parts of your dbt project. Macros are primarily used to encapsulate logic that you need to repeat across multiple models or analyses.
Building Macros with Jinja
Jinja is the templating engine used in dbt that enables the dynamic generation of SQL code. It extends the capabilities of SQL by allowing you to incorporate logic directly in your SQL files through control structures such as loops and conditional statements.
Key Features of Jinja in Macros:
- Control Structures: Integrate conditional logic (if, else) and loops (for) directly within your SQL.
- Variable Interpolation: Dynamically insert values into your SQL code.
- Logic Abstraction: Create complex logic once and reuse it, reducing errors and saving time.
Example; Create a Date Difference Macro
Calculating differences between dates is a frequent need in data analysis for generating features like customer tenure, subscription lengths, or time-to-event data. This macro facilitates easy date difference calculations in various units like days, months, or years.
Here’s how you might use it in a model to calculate customer tenure in days:
Example: Customized Schema Name
In dbt, the generate_schema_name
macro is dynamically used to set the schema name where models are built, using information such as the environment and custom settings from the project configuration. This allows for consistent and customizable schema naming across different deployment environments or organizational requirements.
Here’s an example of a customized generate_schema_name
macro that appends the deployment environment (development, staging, production) to the schema name based on the target environment specified in the dbt project settings:
This macro checks if a custom_schema_name
is provided; if not, it uses the default schema and appends an environment-specific suffix (prod
for production or dev
for other environments). If a custom schema name is provided, it appends this name between the default schema and the environment suffix, ensuring schema names are both descriptive and environment-specific.
Best Practices for Writing Macros
- Maintain Readability: While macros can make your code more concise, always prioritize readability.
Overusing Jinja to abstract SQL can make your models harder for others to understand.
- Document Your Macros: Provide comments within your macro definitions to explain what the macro does, its parameters, and any side effects it might have.
This practice helps others in your team use the macros correctly.
- Organize Macros: Keep your macros organized in a dedicated directory.
Consider naming conventions that make it easy to understand what each macro does at a glance.
- Test Macros: Since macros can be used across multiple models, ensure they are robustly tested to prevent widespread issues in your dbt project.
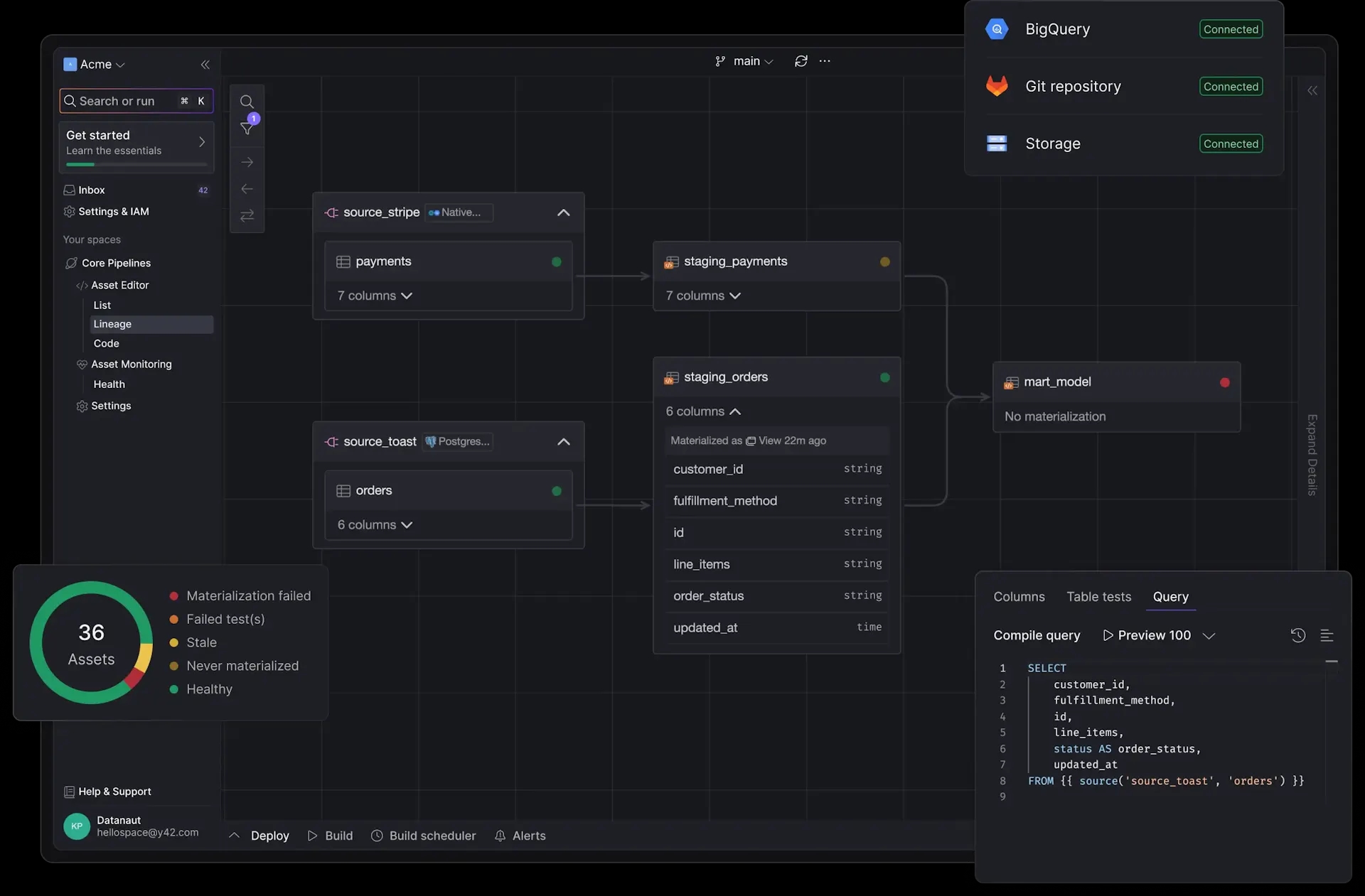
Manage Sources and dbt Models in one place
Build end-to-end pipelines using a single framework.
Get Started