Send Slack notifications
Setting up a Slack Webhook integration
Create a Webhook Integration in Slack
Navigate to the Slack channel where you want to send notifications to. Go to Settings
> Integrations
and create a new automation.
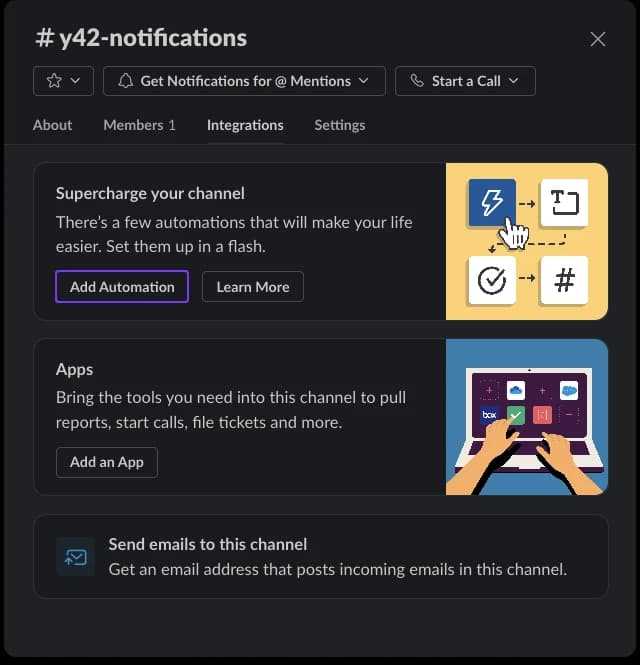
Slack integrations settings.
Add a Webhook and Variables
Select From a webhook
and define variables for your messages. Slack provides an example payload to guide the structure of your request.
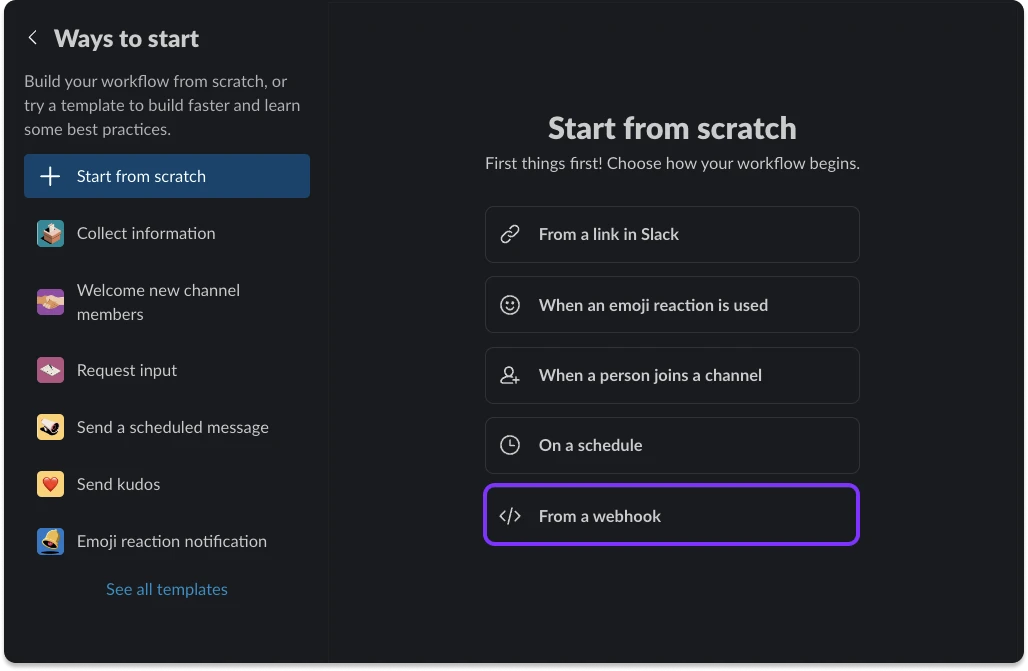
Slack integration webhook configuration.
Compose Your Message
Choose the option to send a message to a channel. Use the previously defined variables to create dynamic messages.
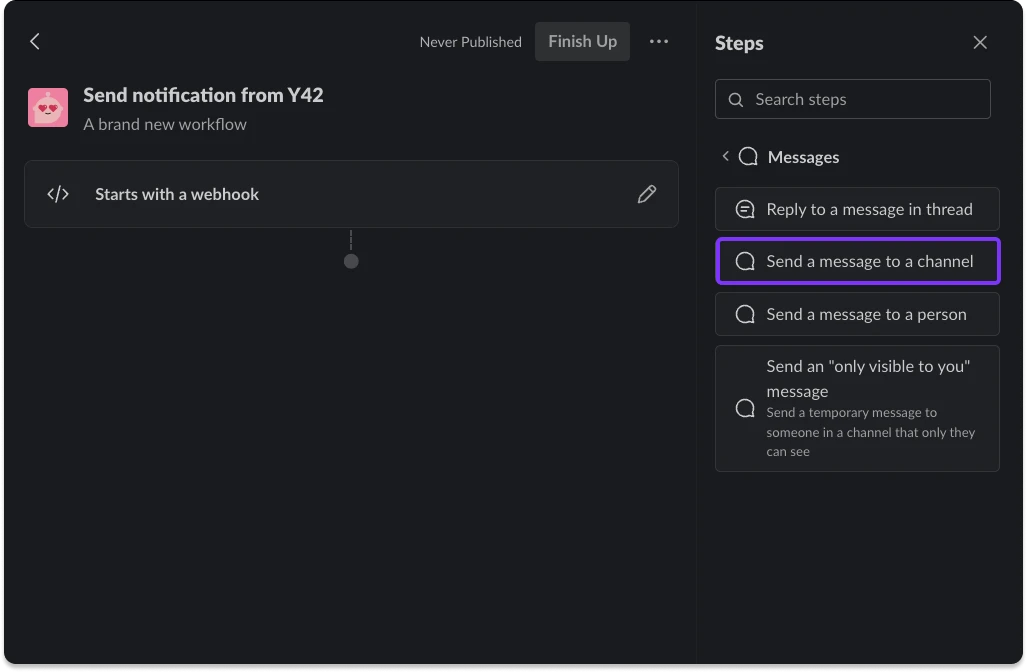
Slack message definition user interface.
Copy the Webhook URL
Access this by selecting the <> starts with a webhook
step. You will use this URL in subsequent steps to integrate with Y42.
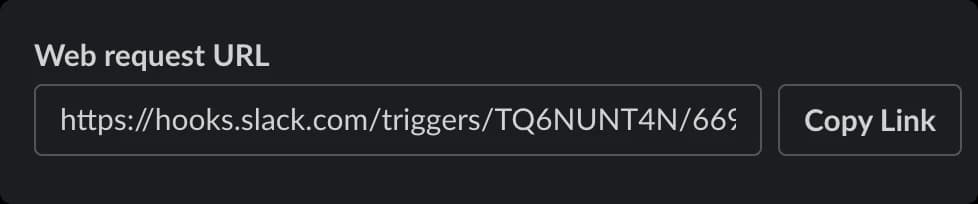
Slack web request URL link with copy button.
Create a Python Action for Slack notifications
Create a Python Action asset with the following script:
This script triggers when the mrt_completed_orders
asset successfully executes.
Ensure dependencies (i.e. assets refereneced in the script) are synced and included in the Y42 exposure file.
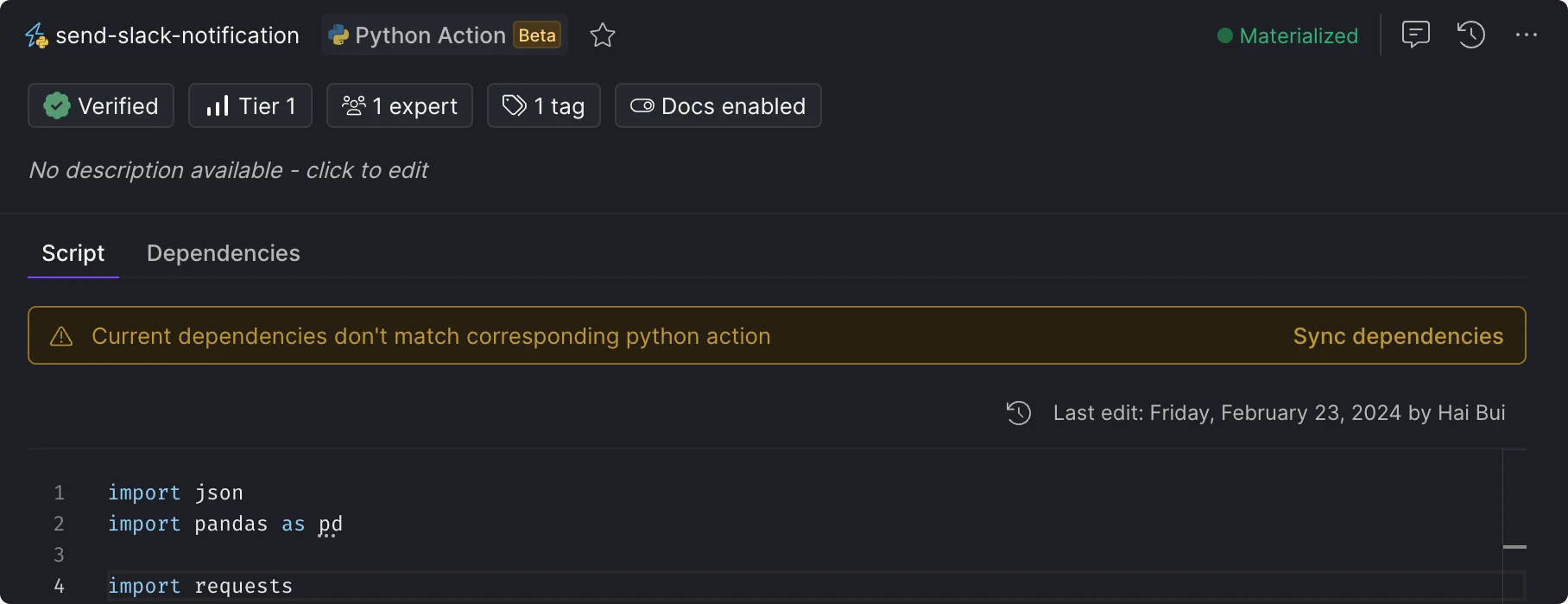
Sync dependencies
Commit changes & trigger the sync
Save your changes by committing them. You can build the asset using dag selectors or via the Build history
tab.
The +
selector, when used in front of the asset name in the command, also triggers all upstream dependencies.
For comprehensive guidance on how to set up Python Action assets, including secret management, check our main documentation page on Python action assets.